Blending Transactions
Blending Transactions are the setup of the algorithm for how Blended Transactions are processed.
Blending Transactions Features
Blending Transactions are a powerful tool in the Financial Services engine that lets you create custom algorithms for automating the creation of multiple Transactions at once according to your custom rules.
When you run a Blended Transaction the algorithm you select to run is a Blending Transaction which holds all the configuration to process it.
Blending Transactions have 5 core sets of functionality to them that make up a Blended Transaction Process. They are as follows:
- Source
This is the source set of Instruments or Transactions the Blended Transaction will use as input to your algorithm - Target
This is the possible ways in which the system will use the source Transactions to create new Transactions automatically against the target. These Targets can be the following:- Instruments
Indicates the system will create new transactions against the specified set of Target instruments. The values will be allocated proportionally across those Instruments according to your Allocation setup - Source Instruments
This indicates for every instrument in the Source, the system will create a new transaction against that instrument. In other words the algorithm is effectively running for every instrument in the Source in an isolated manner. - Allocate From Rule
This can only be used when selecting a source of a Transaction.
That transaction will create new transactions against the entities in the rule using the allocations specified in the rule. - Replicate From Rule
This can only be used when selecting a source of a Transaction.
That transaction will create new transactions against the entities in the rule that are essentially copies of the original transaction. In other words the amounts aren't proportionally allocated they are copied for each entity. - Path
This can only be used when selecting a source of a Transaction.
This will create a new transaction on every entity in the path moving upwards - Path Inverted
This can only be used when selecting a source of a Transaction.
This will create a new transaction on every entity in the path going downwards
- Instruments
- Values
These are values that are aggregated across the source or target transactions that can easily be used in the algorithm itself - New Values
This holds the new values that should be created for each newly created Transaction.- Aggregates
These are the values to provide for the new transaction - Allocations
This is how the values should be allocated across multiple transactions
- Aggregates
- Algorithm
This is the algorithm you can use for creating new transactions automatically. The default algorithm "Value Allocation" allocates the values from the source according to the setup of the Blended Transaction and any custom formulas. You can however create you won Algorithm, see our API for more information.
Algorithms
Blended Transactions can run completely custom algorithms enabling you to perform any operation you want. You can simply create an algorithm by inheriting LemonEdge.API.Processors.Transactional.BlendTransactionProcessorExtender and implementing its ProcessAlgorithm method. See our API for more information on how to create you own custom Blended Transaction Extenders.
The default algorithm, Value Allocation Algorithm, covers most of the functionality you're likely to need when creating Blended Transactions. It provides the ability to automatically create specified Transactions with specified values against sets of Instruments, Paths or Rules, according to allocations you can easily configure. You also have the ability to create formulas against any values for advanced customisation of the functionality.
Value Allocation Algorithm
The Value Allocation Algorithm is the default Blended Transaction algorithm that allows a wide range of use cases for how to configure your algorithms. This can create simple algorithms that allocate underlying transactions to other entities proportionally on a LTD or other basis. It can also create custom algorithms such as FX Revaluation processes.
The Value Allocation performs the following process depending on it's configuration:
- Configured with Source Instruments and Target Instruments
- Here the Source set of Instruments is different to the Target set of Instruments, the user selects both.
- Creates a new Transaction on each Target Instrument for each set of dates.
- By default this will be one set of dates, a LTD calculation or one with a start and end date
- The dates can be setup to repeat, in which case when you specify a start and end date that will be broken down into sets of dates with a start/end according to the repeat period. For instance if repeating every month a start/end date of 1 Jan 2020 to 31 Mar 2020 would effectively run the algorithm 3 times, one for each date set of 1 Jan 202 - 31 Jan 2020, 1 Feb 2020 - 28 Feb 2020, and 1 Mar 2020 - 31 Mar 2020.
- Takes the aggregate values from the Source Instruments on either a LTD basis or just for the specified Start-End dates
- Uses the Allocations specified from the Target Instruments as the mechanism to allocate those values across the Target Instruments
- For each new Transaction against a Target Instrument allocates the required proportion to from the calculated Allocation
- If calculated on a LTD basis then the amount for the new Transaction will be a delta from the calculated total amount for that Instrument - it's current total amount.
- Applies any rounding to the Instrument with the largest allocation
- Configured with Source Instruments and Target "SourceInstruments"
- Here the Source and Target are the same set of Instruments
- For each Instrument it creates a new Transaction for each set of dates
- Takes the aggregate values from the Source Instruments on either a LTD basis or just for the specified Start-End dates
- Sets the values for the new transactions equal to the specified calculated values (usually involving configured formulas)
- Applies and rounding to the Instrument
- Configured with Source Transaction, and Target AllocateRule / ReplicateRule / Path / PathInverted
- Here the Source is a single Transaction (or set of Transactions, such as a Blended Transaction itself)
- The target is an allocation to either a set of entities using an Allocation Rule, or entities along an Allocation Path.
- Takes the aggregate values from the Source Transaction (or set of Transactions)
- Creates a new Transaction with an Entity for that Transaction coming from either an Allocation Rule or Allocation Path.
- The Rule or Path determines the proportion that Entity gets of the Source Values
- In the case of Replication there is no Allocation, and each new transaction receives the same values
The Value Allocation is a simple all purpose algorithm that massively simplifies creating custom algorithms for automatically creating large amounts of Transactions throughout the system. These algorithms can easily configure typical processes such as Hedging, Auto-NAV and other operations.
FX Revaluation Example
You can also use the Value Allocation algorithm with a simple formula to configure normal accounting processes such as FX Revaluations.
For instance if you've configured the following Blending Transaction setup:
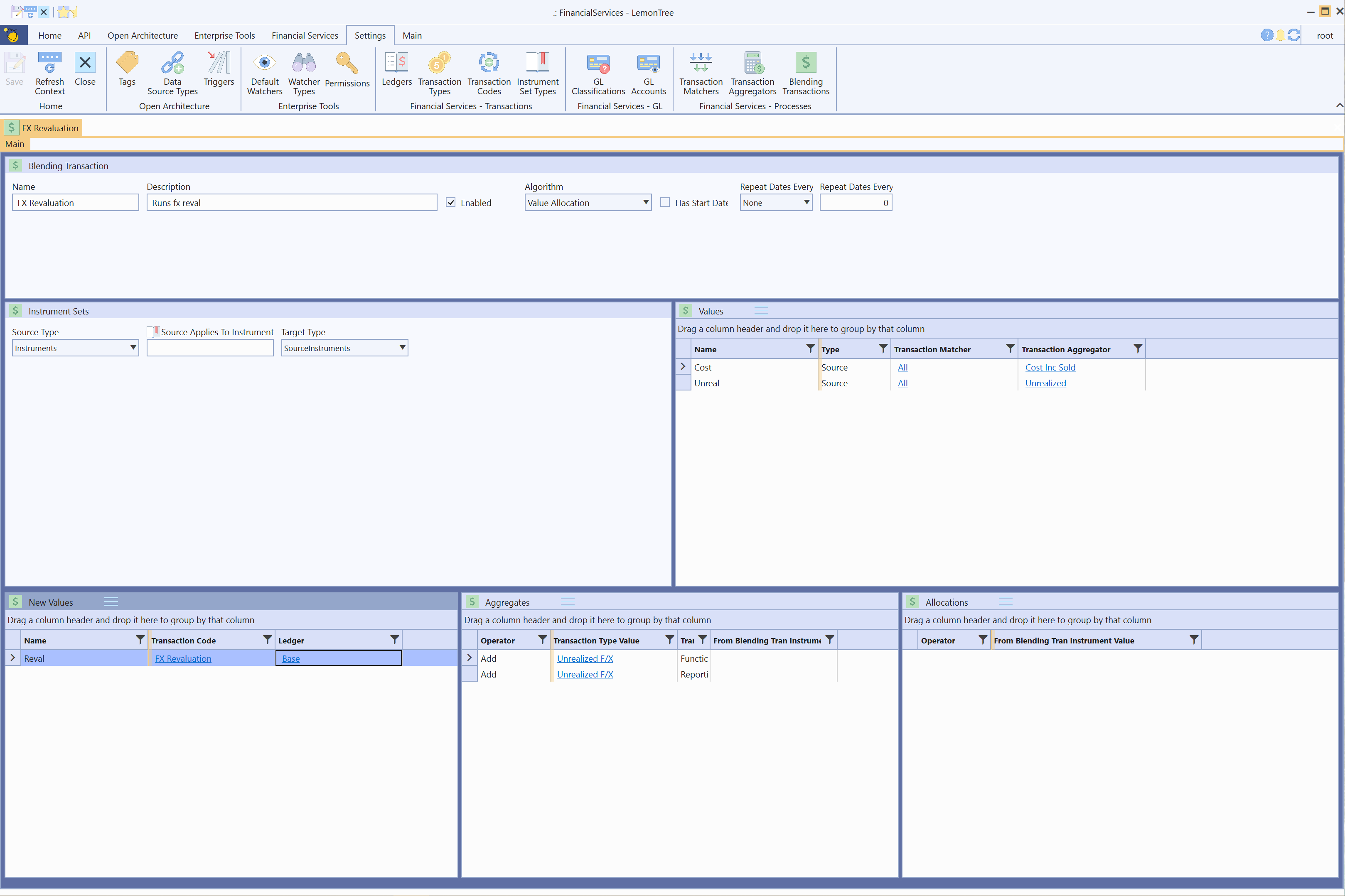
Then we can specify a formula to calculate the Unrealized F/X in Reporting currency like so:
See here for more information on using the Formula Builder.
By having a fully capable Formula Engine you can create simple one line functions, or scripts like the above to step through several processes. Either way you can configure complex processes without having to write your entire algorithm from scratch.
You can of course, always use our API and create your own complete algorithm for intensive complex processes, either way Blended Transactions can cater to your needs.
Views
Blending Transactions configuration use the following views:
Not all of these views are required depending on the Algorithm you are using and what it needs to processes a Blended Transaction.